Hello Everyone,
This is a brief description about angular js.
Now lets start how to work with Angular js in SharePoint 2013 with Rest API.
Step 1: Create a Sample Custom List in SharePoint 2013 . Name it as "EmployeeList"and
create Columns as "Employee","Company","Department".
Step 2: Create a Site Page ,after creating the page Go to
Site Actions-->Edit Page--> Insert -->Web part-->
Media and Content (From categories)-->Select Script Editor -->Add to Page.
Step 3 : Now Copy the below Script and paste it in the script editor by clicking on
the "Edit Snippet" button.
********************************************************************
Now we can see the output for the above script.
This is how it looks like after we fetch the data from SharePoint List ("EmployeeList").
Here the points we have to note is
1. we are not opened server or Connected to server.
2. Not even opened Visual Studio .
3. Not even wrote a single line of SharePoint Server Side Code .
But we have achieved " getting List items from list ". That is the power of Client Object model in SharePoint. Similarly we can achieve lot many functionalities.
Now we can go ahead and look into the script .
****************************************************************
//This section is to Integrate angular JS file into the script (reference file ).
we can also save the files in Style library and also share the file paths here instead of fetching from online .
********************************************************************
var myAngApp = angular.module('SharePointAngApp', []);
Now we will know about an Angular Module. You can think of a module as a container which wires the different parts of your application like controllers, services, directives, etc. To create an Angular Module, write the following code in our SharePointListModule.js file –
*******************************************************************
myAngApp.controller('spCustomerController', function ($scope, $http) {
$http({
method: 'GET',
url: _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getByTitle('EmployeeList')/items?$select=Title,Employee,Company,Department",
headers: { "Accept": "application/json;odata=verbose" }
}).success(function (data, status, headers, config) {
$scope.customers = data.d.results;
}).error(function (data, status, headers, config) {
});
});
We will know adding a Controller which will fetch the data from our SharePoint List using $http service. We will use the SharePoint OData query and bind the data in our user control. Let’s write some code in Customer Controller.js file as shown here
***************************************************************************
This is the detailed description about how to fetch data from SharePoint list using Angular Js in SharePoint Using Rest API.
Hope you like this post....
Thanks for reading...
In this Post , I would like to describe about Angular.Js and how we can use in SharePoint 2013 with Sample example.
With SharePoint 2010, developers were now able to use Client Side Object Model to develop Web parts.The Client Side Object Model is a subset of the SharePoint API which mimics a large portion of the server-side API.The advantage of the client-side API is it can be called from .NET apps, Silverlight or using JavaScript libraries.
In SharePoint 2013, the client-side API has been extended with REST/OData endpoints.
Web Programming world is now shifting its gears towards scripting and there are many frameworks evolved recently all focused on the Model – View * pattern.
I used REST API to talk to SharePoint and get the data from the list.
Lets start about angular JS.
AngularJS :
AngularJS is an open source MV* JavaScript framework maintained by Google and community, for building rich, interactive, single page applications (SPA). AngularJS uses HTML as your template language and lets you extends the HTML vocabulary for your application. The * in AngularJS represents MVC, MVVM or the MVP pattern which makes both development and testing of AngularJS applications easier. The main components of AngularJS are shown here –
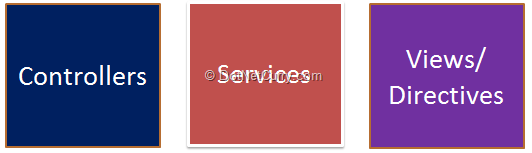
Controller – Controller is key to AngularJS. A Controller is a JavaScript constructor function that is used to create a scope. A controller is attached to the DOM via ng-controller directive. You can use controllers to initiate the state of the $scope object and add behaviours to $scope object. $scope – is a glue between a Controller and DOM and just watches the changes to models and also applies changes to the model. One thing to note about Controller is they do not perform DOM manipulations or maintain state. AngularJS uses Services to maintain state.
Services – Services are used to perform common tasks on the web applications which are consumed by Angular JS via Dependency Injection. Services are registered with a Module and are usually singular to the application.
Views – Views are complied DOM of Angular JS. A view is produced using $compile with HTML templates and $scope.
With client side implementation, Angular can have integration with the server using REST and $http service.
This is a brief description about angular js.
Now lets start how to work with Angular js in SharePoint 2013 with Rest API.
Step 1: Create a Sample Custom List in SharePoint 2013 . Name it as "EmployeeList"and
create Columns as "Employee","Company","Department".
Step 2: Create a Site Page ,after creating the page Go to
Site Actions-->Edit Page--> Insert -->Web part-->
Media and Content (From categories)-->Select Script Editor -->Add to Page.
Step 3 : Now Copy the below Script and paste it in the script editor by clicking on
the "Edit Snippet" button.
********************************************************************
<style>
table, td, th {
border: 1px solid green;
}
th {
background-color: green;
color: white;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.0.1/angular.min.js"></script>
<script src="http://code.jquery.com/ui/1.10.3/jquery-ui.min.js"></script>
<script>
var myAngApp = angular.module('SharePointAngApp', []);
myAngApp.controller('spCustomerController', function ($scope, $http) {
$http({
method: 'GET',
url:
_spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getByTitle('EmployeeList')/items?$select=Title,Employee,Company,Department",
headers: { "Accept": "application/json;odata=verbose" }
}).success(function (data, status, headers, config) {
$scope.customers = data.d.results;
}).error(function (data, status, headers, config) {
});
});
</script>
<h1> Visit SharePointKicthen.com for more Posts.. !!</h1>
<div ng-app="SharePointAngApp" class="row">
<div ng-controller="spCustomerController" class="span10">
<table class="table table-condensed table-hover">
<tr>
<th>Title</th>
<th>Employee</th>
<th>Company</th>
<th>Department</th>
</tr>
<tr ng-repeat="customer in customers">
<td>{{customer.Title}}</td>
<td>{{customer.Employee}}</td>
<td>{{customer.Company}}</td>
<td>{{customer.Department}}</td>
</tr>
</table>
</div>
</div>
***************************************************************Now we can see the output for the above script.
This is how it looks like after we fetch the data from SharePoint List ("EmployeeList").
Here the points we have to note is
1. we are not opened server or Connected to server.
2. Not even opened Visual Studio .
3. Not even wrote a single line of SharePoint Server Side Code .
But we have achieved " getting List items from list ". That is the power of Client Object model in SharePoint. Similarly we can achieve lot many functionalities.
Now we can go ahead and look into the script .
****************************************************************
<style>
table, td, th {
border: 1px solid green;
}
th {
background-color: green;
color: white;
}
</style>
///This section is for displaying the Table data in UI
******************************************************************
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.0.1/angular.min.js"></script>
<script src="http://code.jquery.com/ui/1.10.3/jquery-ui.min.js"></script>
we can also save the files in Style library and also share the file paths here instead of fetching from online .
********************************************************************
var myAngApp = angular.module('SharePointAngApp', []);
Now we will know about an Angular Module. You can think of a module as a container which wires the different parts of your application like controllers, services, directives, etc. To create an Angular Module, write the following code in our SharePointListModule.js file –
*******************************************************************
myAngApp.controller('spCustomerController', function ($scope, $http) {
$http({
method: 'GET',
url: _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getByTitle('EmployeeList')/items?$select=Title,Employee,Company,Department",
headers: { "Accept": "application/json;odata=verbose" }
}).success(function (data, status, headers, config) {
$scope.customers = data.d.results;
}).error(function (data, status, headers, config) {
});
});
We will know adding a Controller which will fetch the data from our SharePoint List using $http service. We will use the SharePoint OData query and bind the data in our user control. Let’s write some code in Customer Controller.js file as shown here
***************************************************************************
In the script we just saw, we have first created an Angular Controller with the name ‘spCustomerController’. We have also injected $scope and $http service. The $http service will fetch the list data from the specific columns of SharePoint list. $scope is a glue between Controller and a View. It acts as execution context for Expressions. Angular expressions are code snippets that are usually placed in bindings such as {{ expression }}.
You might have observed that we are creating separate JavaScript files for creating Angular JS Module and Controller. As already mentioned, the AngularJS module acts as a container for Controllers, Services, Filters, and Directives etc. Modules declaratively specify how an application should be bootstrapped. It offers several advantages like –
- The declarative process is easier to understand
- Modules can be reusable
- Modules can be loaded in any order or parallel
- Unit testing and end-to-end testing is easier
This is the detailed description about how to fetch data from SharePoint list using Angular Js in SharePoint Using Rest API.
Hope you like this post....
Thanks for reading...
Works like a charm :)
ReplyDeleteCan we have a downlaod button to export all data to excel in zip format.
thanks for ur words..:-)
Deletepls check the foll'g post..
http://sharepointkitchen.blogspot.nl/2015/01/export-html-table-to-excel-by-using.html
Thank you for your information.
ReplyDeleteYii Development Company India
Thanks...
DeleteCan you provide solution for updating selected list item from above rendered table?
ReplyDeleteYeah.. Will try and update the same in blog
Deleteinteresting blog. It would be great if you can provide more details about it. Thanks you
ReplyDeleteYii Development Company India
It is not working only header with one row displaying {{customer.Title}},{{customer.Employee}},{{customer.Company}},{{customer.Department}}
ReplyDeleteNice Blog , This is what I exactly Looking for , Keep sharing more blog .
ReplyDeleteremote yii framework developers
This post is more informative. Thanks for sharing this valuable information.
ReplyDeleteLeadership Programs
Management And Leadership Training